Arrays in Java
In the previous blog, we learned about the Control Flow in Java. If you want to know more about it, visit the Loops in Java. In this blog, we will go through the concept of Arrays in Java. We all are already familiar with Arrays as we have learned them in C and C++, same is applicable in Java. It is the collection of the same types of data that stores the elements in a contiguous manner. Contiguous is nothing but the elements are stored in a sequential manner i.e. 1st element on the 0th index position, 2nd element on the 1st index position, and so on.
Declaration of An Array
The elements in an array can be accessed using an index of integer type. For e.g. suppose we have an array named arrayValue which is an integer array, then arrayValue[i] is an ith integer in the array. Coming to the declaration of the array, we declare an array by specifying the data type, followed by the variable name and [ ].
e.g.
In the above example, we have only declared the array variable. It is not yet initialized. In order to initialize it, we use a new operator.
e.g.
The length of an array int[50] is not constant, it can vary as per the requirement. Once the array is created, we cannot change the length of the array. If there is a need to frequently change the array, then we should use an array list in that case. We will cover the array list later. In Java, there is a shortcut where we can do both declaration and initialization without using a new operator.
e.g.
When we initialize the array, it is mandatory to place a comma after each element, and the comma after the last element is allowed, so that whenever we want to add the other element we can add after it.
We can also create anonymous arrays. Anonymous means we can create an array without giving names.
e.g.
In the above expression, a new array is allocated and filled with the values within the braces(). The array size is set accordingly depending on the count of the initial values. We can even use this syntax for reinitializing an array rather than creating a new variable.
e.g.
Accessing Array Elements
As we have already seen that array stores the elements in a sequential manner, so the array elements start from 0 to nth. Once the array is created, we can fill the elements in an array by using a loop.
e.g.
When we create an array, every array has a default value based on their data types, e.g. for the number all the elements in an array are initialized with 0. The boolean array is initialized to false. Arrays of objects are initialized by null values, which means that they don’t hold any objects.
e.g.
The above statement will create an array of 5 strings, each of which will be null. Suppose we want that the array should hold the empty string, then we must supply them " ".
e.g.
Suppose we want to find the length of an array (number of elements in an array) then we should use array.length
e.g.
Copying Array
In order to copy the array variable into another, then it is must that both the variables should point to the same variable.
e.g.
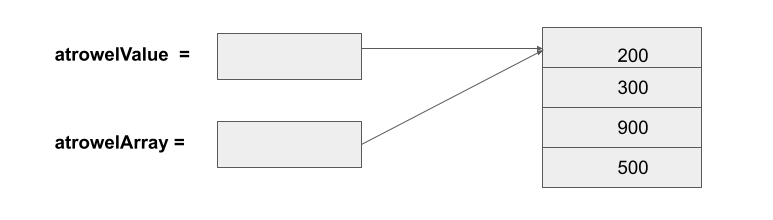
Suppose in case we want to copy all the elements of an array into a new array, then we can use copyOf() method of Arrays class.
e.g.
The first parameter is the new array and the second parameter is the length of the new array. This method can be used to increase the size of an array. The elements that are additional in an array can be filled with 0 if the array has a number in it, and false if it has boolean values.
Command Line Arguments
There are times when we want to provide input through a command prompt, then in that case use command line arguments. We have used String args[] in the main method in a number of our examples. String args[] is nothing but the arguments which are specified on the command line.
e.g.
Compile:
Output:
Array Sorting
In order to sort the array of numbers, we can use one of the sorting methods of an array.
e.g.
sort() uses the version of one of the sort i.e. Quick Sort which is very efficient for data sets.
e.g.
Output:
Multidimensional Array
We use a multidimensional array for accessing the elements of an array that uses more than one index. Suppose we want to make a table of the marks that students have secured in the subjects like C, C++, Java, etc.
Names of Students | C | C++ | JAVA |
---|---|---|---|
John | 58 | 65 | 76 |
Sam | 43 | 68 | 69 |
In order to store the above information, we will need a 2-dimensional array called marksScored. Declaring a 2-D array is not so difficult. We just have to declare a variable with the data type we require along with the bracket[ ][ ].
e.g.
We cannot use an array until we have initialized it. The initialization is as follows:
Suppose we know the array elements in the array, then we can use a shorthand notation for initializing array elements, rather than using a new operator.
e.g.
Once we have initialized an array, we can access the individual elements by providing two pairs of brackets i.e. marksScored[ ] [ ].
Now suppose we want to print the elements of an array, then we can use the following statements.
e.g.
Output: