Exceptions in Java
In the previous blog we learned about the Inner Classes in Java. If you want to learn about the Inner Class then visit Inner Classes in Java . In this blog, we will go through Exceptions, Assertions, and Logging. Exceptions are unwanted or unexpected events that occur during a program's execution, i.e. at run time, and interrupt the normal flow of the program's instruction. Exceptions are caught and handled programmatically. It is not every time that our code works as expected without any bugs in it. There are times when our code has bugs or some external circumstances due to which it may be possible that the user may lose the work which he did during that particular session, in this case, the user will never visit our application. To overcome this situation Java has introduced the concept called exception handling. We will go through it in detail in the following sections:
Dealing with Errors in Java
Let's consider an example if our program is running and suddenly an error occurs and that error is caused due to wrong information contained in a file or network connection issues or invalid array index or object references. The user thinks or expects that the program will still run when the error occurs. If the operations remain incomplete due to the error then the program must return to the safe state and enable the user to perform other commands or the user should be allowed to save all his work and exit without any issues. So to deal with this situation Java has introduced exception handling wherein its mission is to transfer the control from where the error occurred to an error handler that can deal with the situation. We must take into consideration the errors that may occur when we are running our program
- User Input errors
- Device errors
- Physical limitations
- Code errors
Exception Hierarchy in Java
In Java an exception object is an object of the class derived from Throwable. We can even create an exception if the requirements are not fulfilled by the built-in exceptions.
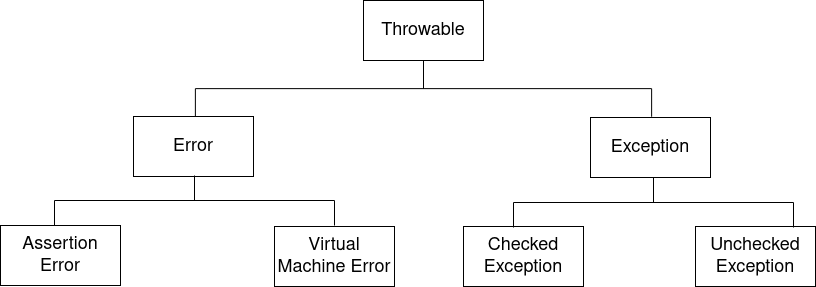
Types of Exceptions
Following are the types of Exceptions:
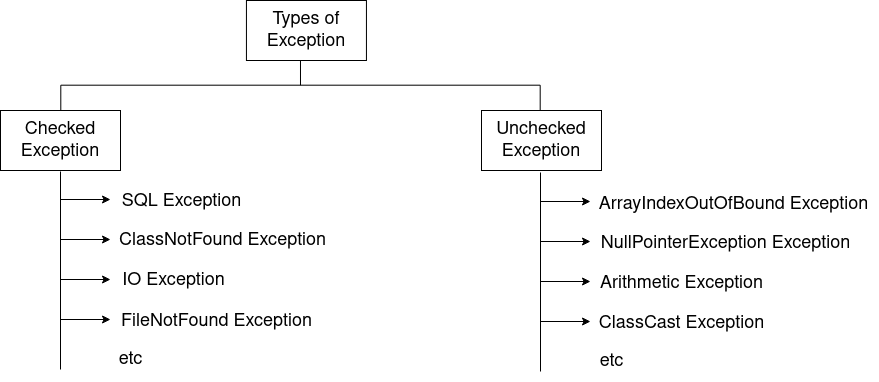
There are two types of Exception
- Checked Exception
- Unchecked Exception
- Checked Exception
- Unchecked Exceptions
Checked Exceptions are the exceptions that are checked by the compiler during compile time. These exceptions cannot be ignored. We must handle them otherwise the program gets terminated if these exceptions occur.
e.g.
Output
Unchecked Exceptions are the exceptions that occur at the time of execution. So these exceptions are called Runtime exceptions. These exceptions occur due to logic errors, improper use of API, etc. The compiler ignores this exception at compile time.
e.g. Example of ArithmeticException
Output
e.g.Example of NullPointerException
Output
e.g. Example of ArrayIndexOutOfBoundException
Output