Inner Classes in Java
In the previous blog, we learned about the concepts which were remaining from Lambda Expression in the Java blog. If you want to go through the previous blog click Lambda Expressions in Java - Part 2 In this blog, we will go through the concept of Inner Classes in Java. The Inner Class is nothing but the class which is defined inside the other class. There are two reasons for using the Inner Class:
- Inner Class can access all the members of the outer class including private members.
- Inner Class can be hidden from the other classes that are defined in the same package.
Syntax of Nested Class
In the above syntax OuterClass is nothing but the class which holds the inner class and the InnerClass is the class that is written in the other class. The Nested Class is broadly divided into two types:
- Static Nested Classes
- Non-static nested Classes
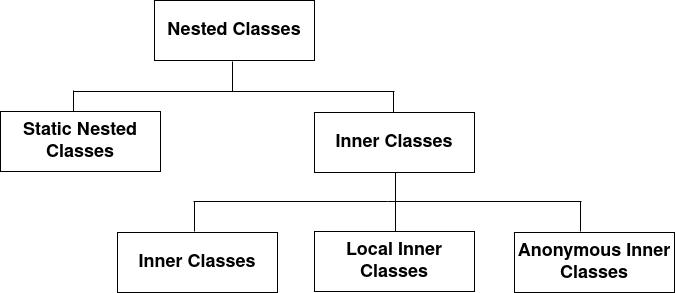
Non-static nested Classes(Inner Classes)
We cannot define a class with a private access modifier because defining a class as private will restrict the methods and fields from accessing its own class. In order to overcome this the class can be defined as a member of another class, so that the inner class can be made private. With the help of this, we can also access the class's private members. Non-static nested Classes are broadly divided into three types:
- Inner Classes
- Local Inner Classes
- Anonymous Inner Classes
- Inner Classes
- Local Inner Classes
- Anonymous Inner Classes
Inner Classes can be created easily. Inner Class is nothing but the class which is written within another class. We can declare the inner class as private. Once the class is declared as private the class cannot be accessed from the object outside the class.
e.g.
Output
In the above code we can see that OuterClass is an outer class and the InnerClassEx is an inner class and displayInnerClass() is the method in which we are creating the object of inner class and using that object calling the method of inner class i.e. display() method. The method in which the object of the inner class is created is invoked from the main() method.
We can also access the private method using the inner class. For Example
e.g.
Output
In the above code InnerClassEx class has a method called getValue() which returns the value of the private member and that the class TestInnerClass calls that method defined in the inner class. In the TestInnerClass class, we have created the object of the Outer class and with the help of that object, the object of the inner class is created.
In Java Local Inner Classes are the classes that are created inside the method. As they are created inside the method their definition is inside the method block. This method block can have statements like method definition, for loop, if cause, etc. Local Inner Classes don't have any access specifier because they belong to the block they are defined within. But they can be defined as final or abstract.
e.g.
Output
In the above code OuterClassEx class has a method getValue(). In the body of the getValue() method a class called InnerClassEx is defined and in that class, there is a method named displayValue() which is called using the object of the InnerCassEx class. In the main() method the OuterClassEx object is created and with the help of that object, the getValue() method is called.
We all are familiar with the term anonymous. In general terms anonymous means unnamed or nameless. A similar concept is used in an Anonymous Inner Class. Anonymous Inner Class is an inner class that is declared without the class name. Anonymous Inner Class can be declared and instantiated at the same time. The purpose of using Anonymous Inner Class is to override the method of a class or an interface whenever we want. The general syntax of Anonymous Inner Class is as follows:
Syntax
e.g.
Output
Passing Anonymous Inner Class as an argument to the method
e.g.
Output
Static Nested Class
Static nested class is a class which is a static member of the outer class. As we know static classes don’t have access to their methods or member variables, same is the case with the static nested class they also don’t have access to its methods and member variables of the outer class.
Syntax
e.g.