Data Types in Java
In the previous blog we learned the java shell tool. If you want to know more about it then visit Java Shell Tool(JShell). In this blog, we will go through the data types in Java. In general, variables are used to store the data. Variables are nothing but whose values keep on changing. We will go through Variables in our upcoming blog. While using the variables a question arises what kind of data the variables should store? If the program's data type is not known, it won't be very clear for a programmer to code a program. Hence the concept of Data types is used.
What is a Data type
Before going through the concept of Data types first let's understand what is statically typed language and dynamically typed language? In Statically typed Language, the expression type and variables are known already at compile time. Once variables are declared to be of a particular data type, then they cannot hold the values of different or other data types. On the other hand in Dynamically Type Language, the variable’s type is checked at runtime. Java is a static typed language meaning all the variables in Java must be declared before use.
In the above example, age is a variable that has data type as int. It specifies that the age variable can only have integers.
Now we will go through the types of Data Types:
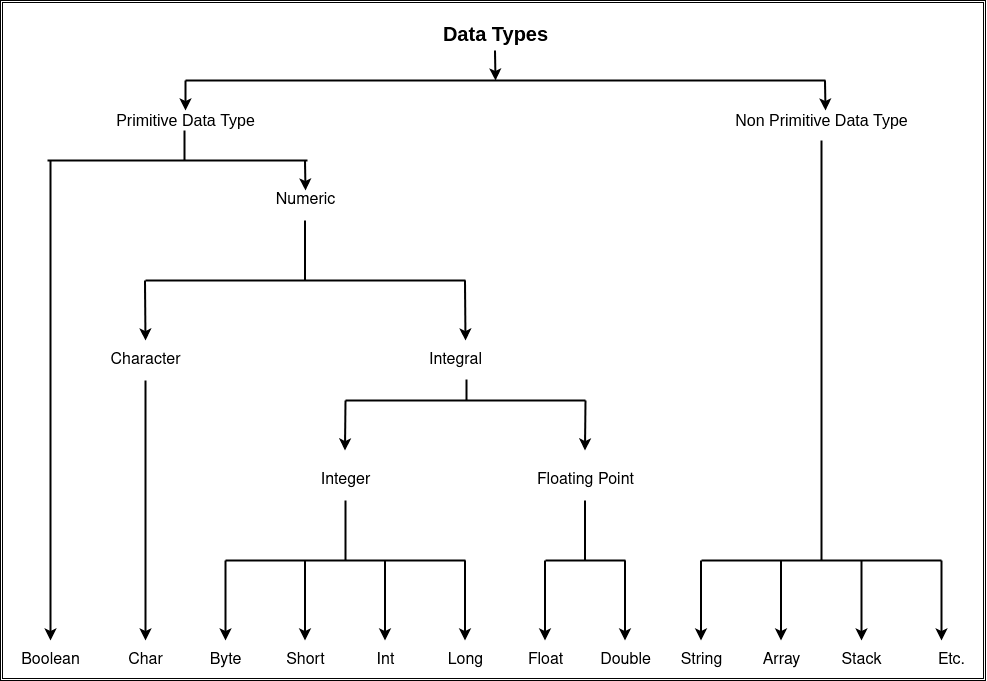
Data types are broadly divided into two types:
- Primitive Data type
- Non Primitive Data Type
Primitive Data type
Primitive data types are the predefined data types of Java that specify the size and the type of any standard values. There are eight primitive data types of which four are Integer type, two are floating type, one is boolean type and the other one is a character type. Types of Primitive Data Types:
boolean
Boolean is used to store two values i.e. true or false. The boolean data type is used as a simple flag to track true or false conditions. It represents one bit of information but its size is not precisely defined.
e.g.
byte
Data type that is an "8 bit" two's complement integer is called a byte. Bytes range minimum value of -128 and a maximum value of 127. If we know that the variable which will be used will be within -128 to 127, then byte can be used instead of int to save memory.
e.g.
short
short is a 16-bit signed 2's complement integer. It ranges from -32,768 to 32,767. Similar to a data type byte, short is also used for saving memory.
e.g.
int
int data type is a "32-bit" signed 2's complement integer, that ranges from -231 to 231-1. If the version of Java is 8 or beyond 8, then we can use an unsigned 32-bit integer which will have a minimum value of 0 and a maximum value of 231-1
e.g.
long
long data type is a "64-bit" signed 2's complement integer, that ranges from 263 to 263-1. In Java SE 8 and later, the long data type can be used to represent a positive 64-bit long, which ranges from 0 to 264-1.
e.g.
float
float is a single-precision (32-bit use for representation of a decimal number, IEEE 754 ) floating-point number. It is recommended to use float instead of double if you want to save memory in large arrays of floating point numbers. Float cannot be used for precise values, such as currency because it will give roundoff errors. The default value of float is 0.0F. We must use the suffix 'f' or 'F' at the end of the value to denote the float data type. It is mandatory to use f or F at the end of the value because if there is no suffix then it will be considered double.
e.g.
double
double is a "double-precision 64-bit" IEEE 754 floating point number. Its value range is almost unlimited. It is generally used for decimal values similar to floats. Just like float, double data type also shouldn't be used for precise values, like currency. The default value of double is 0.0d. It is denoted by the suffix 'd' or 'D'. It is not mandatory to use suffix because by default if there is no suffix in the floating point value then it is considered double.
e.g.
char
char data type is a single "16-bit" Unicode character (UTF-16). It ranges from a minimum value of '\u0000' or 0 to a maximum value of '\uffff' or 65,535 inclusive. The meaning of Unicode is nothing but it represents all characters that exist in world languages. It starts with U+, It is a hexadecimal format, starting from U+0000 to U+10FFFF with supplementary characters.
e.g.
Size of Data type in Java
Data Type | Size | Range |
---|---|---|
byte | 1 byte | –128 to 127 |
short | 2 byte | –32,768 to 32,767 |
int | 4 byte | –2,147,483,648 to 2,147,483,647 |
long | 8 byte | –9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 4 byte | ~7 significant decimal digits |
double | 8 byte | ~15 significant decimal digits |
char | 1 byte | ~15 significant decimal digits U+0000 to U+FFFF (0 to 65,535) |