Java Shell Tool(JShell)
JShell is a tool for learning java. It is an interactive tool. JShell provides a read evaluate print loop (REPL). It evaluates the statements, expressions, etc and displays the result immediately. JShell runs one statement at a time. It runs one statement and prints the output. Due to this we can even do variations in our statements by looking to the previous output. JShell is run from the terminal. Running JShell is very easy, you just have to write jshell in your terminal and JShell will start.
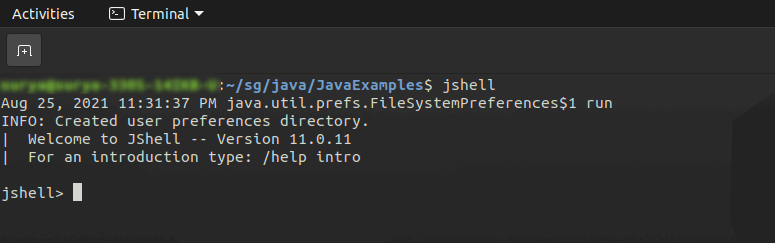
To do code is very easy here. Now suppose if we want to type a string "Java Short Kicks". In Java we had to write a class and main() method, then compile and run, after doing all this then code gets run. But in Jshell you don't have to do this much, you just have to type the string in "".
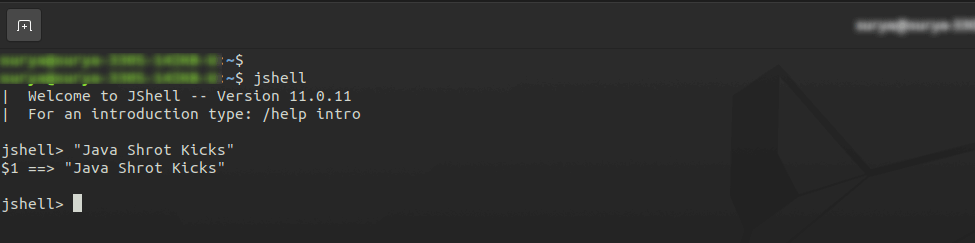
If you want to do calculation, then simply write 3 + 4 it will give you the output.
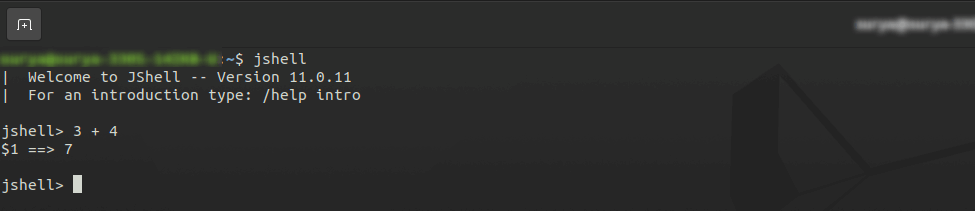
If you want to calculate the length of the string then you can do by the following "Java Shortkicks".length()
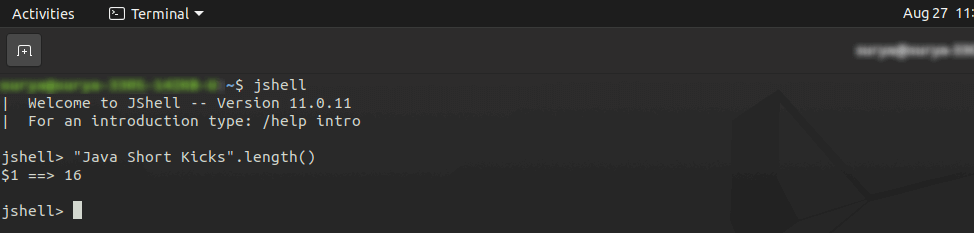
If you what to write some expression and in that expression if you want to use result of the previous statement then you can do it by the following:
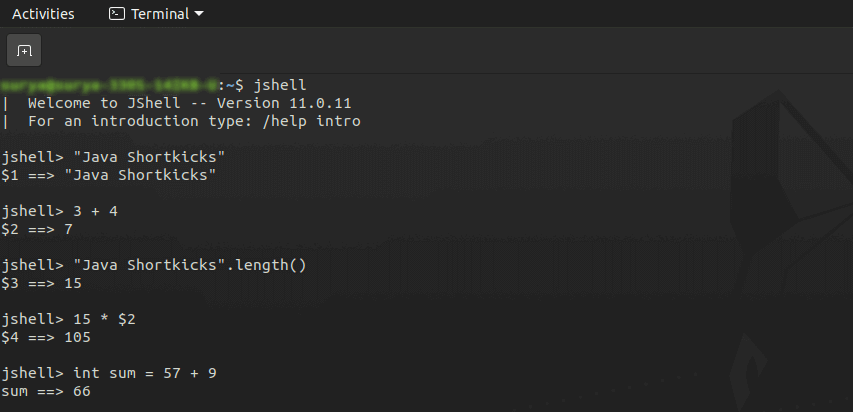
In the above example, $2 is like a location where we have calculated the sum, the above expression will give the result as 105 i.e. we have given the value 15 it is multiplied by the value of $2 i.e. 7. We can also use variables to store the values.
To exit JShell, we need to enter /exit on terminal:
JShell Command's
All Jshell commands start with forward slash(/). JShell provides various commands to update environment, current code variables information, methods information, and types information. Now let's check some basic commands:
/help — Shows commands and their arguments.
/vars — Shows current variables information.
/methods — Shows current methods information.
/types — Shows current types information.
/list — Shows list of entered snippets information.
/import — Shows current import packages.
/list -all OR /list -start — Shows default startup script.
/**Press tab key OR /list -**Press tab key — Tab key shows all possible commands and command arguments.
Command Abbreviations
JShell provide all unique commands abbreviated. For example we can use the /l -a abbreviations to enter the /list -all command.
/se abbreviation for /set.
/he abbreviation for /help.
/op abbreviation for /open.