Concrete Collections in Java - LinkedList
In the previous blog, we learned about Collections in Java. If you want to learn about Collections, visit Collections in Java . In this blog, we will learn about Concrete Collections in Java. Following are the Classes in the Collection framework. We will discover them one by one. We will go through the Concrete Collection named LinkedList. LinkedList stores the object in a separate link. Each link stores a reference to the next link in the sequence.
Collection Framework Hierarchy
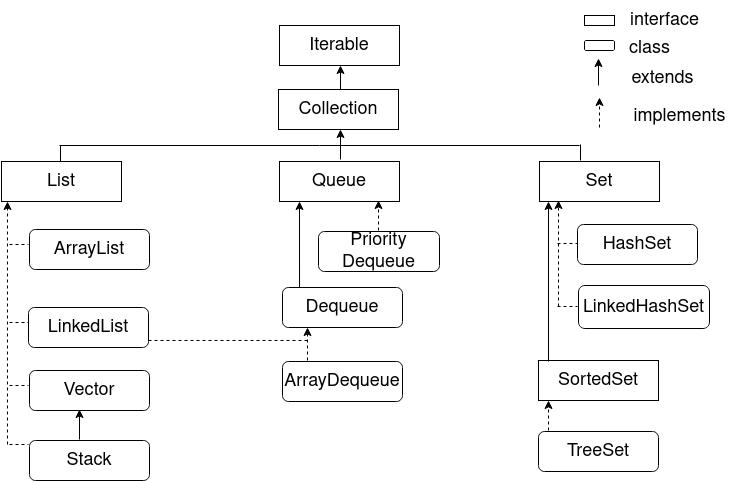
LinkedList
We have already learned about Arrays and ArrayList in our previous blogs. But Array and ArrayList have major drawbacks. The main drawback is, in an array and array list Removing an element from the middle of an array is costly because all array elements beyond the removed one must be moved toward the start of the array. The same is applicable for inserting the elements in the middle. In order to solve this problem Collection Framework has a data structure called LinkedList. LinkedList stores the object in a separate link. Each link stores a reference to the next link in the sequence. All the Linked Lists in Java are doubly Linked i.e. each link in the linked list stores the reference of its predecessor.
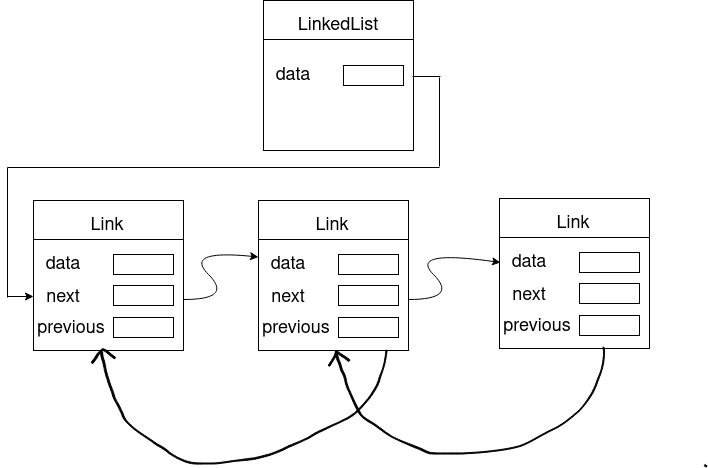
In the above figure, we can three fields i.e. data, next and previous. We will see all 3 fields one by one:
data - It stores the actual data.
next - It stores the address of the next element in the linked list. By default, the value of the first element is set as null.
previous - It stored the address of the previous element in the linked list. By default, the value of the last element is set as null.
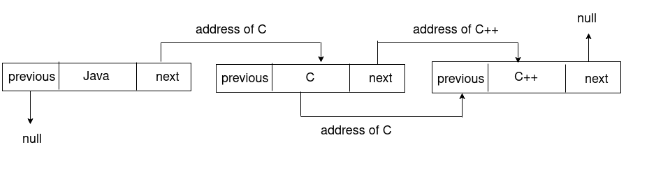
In the above diagram, we can see that the previous field is null and the next field holds the address of the next node data field i.e. C. The next field of the C node holds the address of the data field i.e. C++. The previous field of the C++ node holds the address of the data field of the C node.
Syntax for Creating a LinkedList
Syntax
e.g.
Example of Creating a LinkedList:
Output
Methods of LinkedList
There are several methods in the LinkedList from which we will see the 4 commonly used methods in detail.
- add()
- set()
- get()
- remove()
- indexOf()
- lastIndexOf()
- contains()
- clear()
- iterator()
add() method is used for adding the elements at the end of LinkedList in the linked list.
e.g.
Output
set() method is used for changing the elements of the LinkedList.
e.g.
Output
get() method is used for accessing the elements from the LinkedList.
Syntax
e.g.
remove()method is used to delete or remove the elements from the Linked List.
e.g.
Output
indexOf() method returns the index of the first occurrence of the element.
e.g.
Output
lastIndexOf() method returns the index of the last occurrence of the element and returns -1 if there is no occurrence of that element.
e.g.
Output
contains() method check whether the LinkedList contains the element or not.
e.g.
Output
clear() method removes all the elements from the Linked List.
e.g.
Output
Iterator can be used to loop the LinkedList. If there are more elements in the LinkedList, the hasNext() method returns true, otherwise it returns false. The purpose of the next() method is to return the next element of the linkedList, but if there is no next element then it will throw an exception NoSuchElementException.
e.g.
Output