Loops in Java
In the previous block, we learned about the Control Flow in Java. If you want to know more about it, visit Control Flow. In this blog, we will learn about the loops in Java. We use loops to repeat a block of the program multiple times until the specific condition satisfies. The following are the types of loops:
- while Loop
- do-while Loop
- for Loop
while Loop
while loop executes when the condition in the while statement is true. If the condition of the while statement is false, then the while loop will not be executed. The general syntax of a while loop is:
Syntax
Flowchart
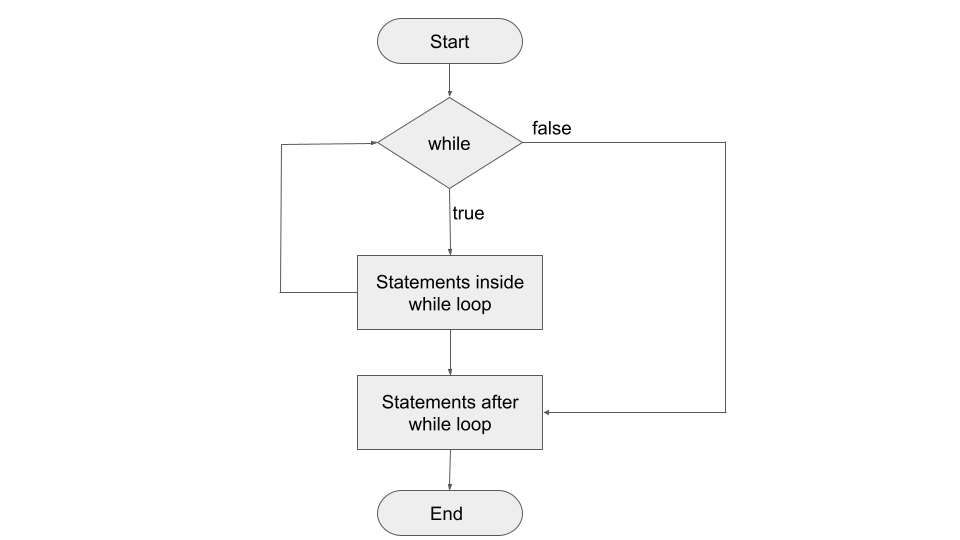
e.g
Output
do-while Loop
There are times when the block should be executed at least once, then we use a do-while loop. It works the same as a while loop, but the only difference is that the block gets executed at least once, even though the condition doesn’t satisfy. The general syntax is
Syntax
In the above statement, the statement will be executed first and then the condition will be tested. If the condition satisfies then it will repeat the statements and retest the condition and so on.
Flowchart
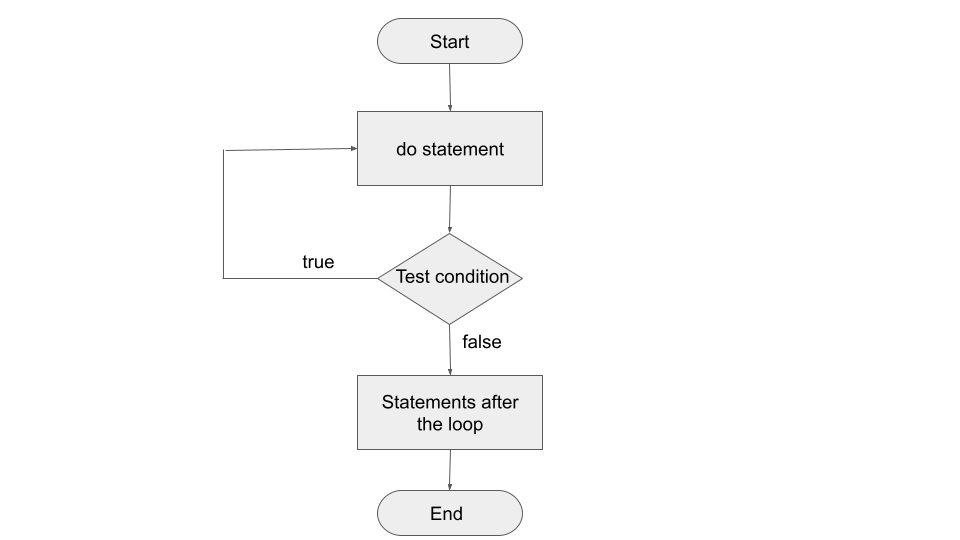
e.g
Output
Now we will see an example where the condition fails and still the block executes once.
e.g
Output
for Loop
for loop is used when we want to execute the statements iteratively for the specific number of times. The general syntax is
Syntax
Here the first part is the initialization of the counter variable. This is the part from where the for loop starts execution. Without initialization, we cannot start the execution of the for loop. The second part is the condition, if the condition is true then it executes the statements inside the for loop. The statements are executed until the condition becomes false. The third part is increment/decrement operation. It increments/decrements the value of the counter variable.
Flowchart
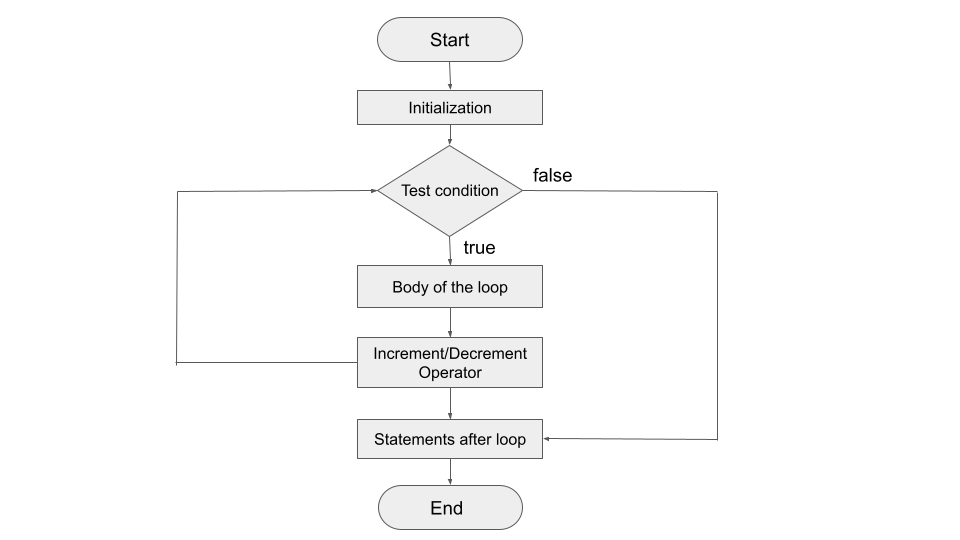
When we declare a variable in the first part of the loop, then its scope is till the end of the for loop. Suppose we want to use the final value of the counter, then we have to declare it outside the loop, because if it's declared inside the for loop, then we cannot use the value outside the loop.
e.g
Output