Map Interface in Java
In the previous blog, we learned about ArrayDeque. If you want to know more about it, visit ArrayDeque in Java. In this blog, we will go through the Map Interface.
Map Interface
Map interface maps the keys with values meaning elements in the Map are stored in key-value pairs. Each key is a unique key associated with individual values. Map cannot contain duplicate keys. Map interface is present in java.util package. A Map is useful when we want to search, update or delete an element on the basis of the key. The examples where Map can be used are:
- A map roll no and students
- A map of zip codes and cities, etc
Syntax of Map
Classes that implements Map interface
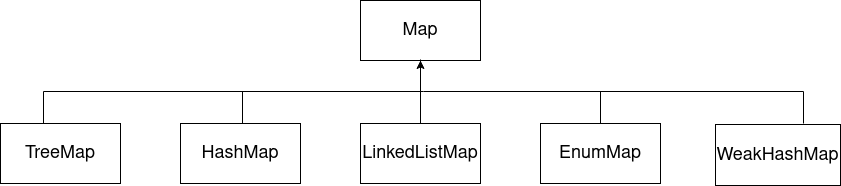
Classes that implements Map Interface are shown above
- TreeMap
- HashMap
- LinkedListMap
- EnumMap
- WeakHashMap
Methods of Map
- put(Object k, Object v)
- size()
- putAll()
- clear()
- get(Object k)
- getOrDefault(Object k, V defaultValue)
- containsKey(Object k)
- containsValue(Object v)
- remove(Object k)
put(Object k, Object v) method is used to insert or add an entry to the Map.
e.g.
Output
size() method is used to get the size of the map which refers to the number of key and value pairs.
e.g.
Output
The putAll() method inserts or adds the specified map to the map.
e.g.
Output
clear() method is used to clear or remove all the elements from the specified Map.
e.g.
Output
get(Object k) method returns the object that contains the value associated with the key.
e.g.
Output
getOrDefault(Object k, V defaultValue) method returns the value to which the specified key is mapped, or if no value is found then it returns defaultValue.
e.g.
Output
containsKey(Object k) method is used to verify or check if the specified key k is present in the map or not.
e.g.
Output
containsValue(Object v) method is used to verify or check if the specified value v is present in the map or not.
e.g.
Output
remove(Object k) method removes the entry from the map represented by the key k.
e.g.
Output