Strings in Java
In the previous blog we learned about the Bitwise Operator. If you want to know more about the Bitwise Operators then visit Bitwise Operators in Java. In this blog, we will go through the basic concept of Strings in Java and its methods. In Java String is a sequence of characters i.e. the character array. There is no built-in type for string. Rather, the Java library contains a predefined class which is called String. String class is immutable meaning once created we cannot change it, if we change it, then it will create an entirely new String. In order to create a String there are two ways:
- Using String Literal
- Using new keyword
Using String Literal
String can be created using the literals
e.g
The string which will be written in double quotes will go to one of the two places i.e. String constant pool or heap. For example in the above code the string “Welcome to Strings in Java Blog” will be stored in the string constant pool. JVM will then check for the existence of the string, if the string exists then it will reference the instance of that string.
e.g
In the above example stringMsg contains a String "Welcome to Strings in Java Blog", so "Welcome to Strings in Java Blog" will be stored in string constant pool and stringMsg will be pointed to it. In the second line as the stringMsg2 has the same String, so here stringMsg2 will point to the same location in the String Constant Pool where "Welcome to Strings in Java Blog" is stored.
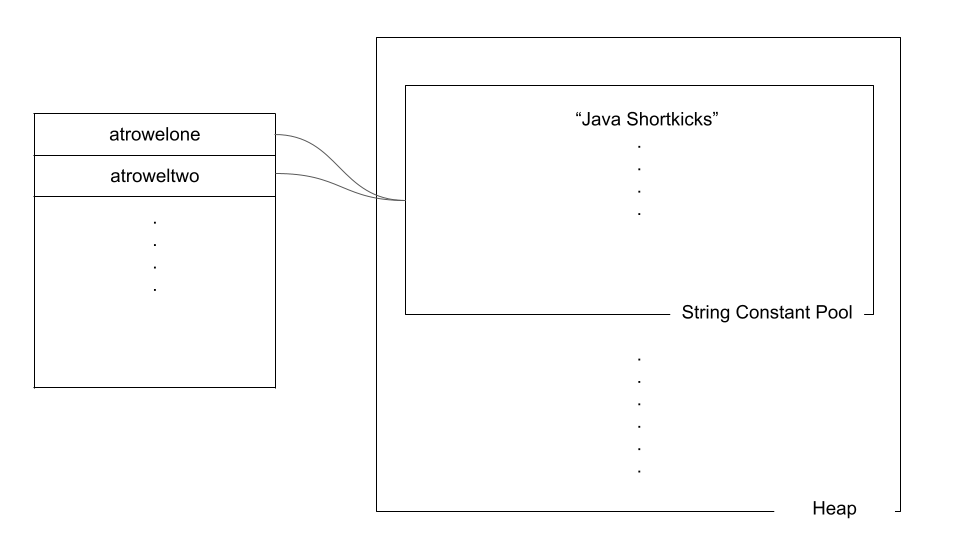
Using new keyword
String can be created using the new keyword
e.g
Now here, JVM will create a new string object in the heap and will store the literal "Welcome to Strings in Java Blog" in a heap and the variable stringMsg will be pointing to the location where the string “Welcome to Strings in Java Blog” is placed.
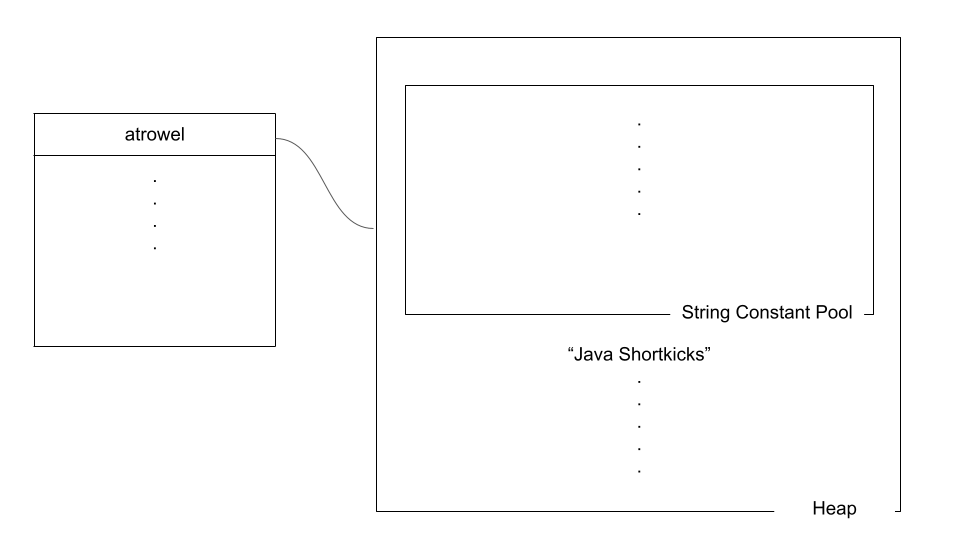
Methods of String in Java
charAt()
If we want to know what is the character value for the particular index in the string then we can use charAt(). The index starts from 0 to n-1, just like array indexing.
Syntax
e.g
Output
Here in the example we want to find out which character is at the 11th location then, the count will start from 0 and will end with n - 1. The string contains 30 characters including a blank space in the middle, so the last character value will be 29(n-1 i.e. 30 -1). We cannot give an index greater than the string length, otherwise it will return an exception StringIndexOutOfBoundsException.
e.g
Output
compareTo()
compareTo() lexicographically compares two strings. The comparison is predicated on the Unicode value of every character within the strings. It compares the sequence of characters in the given string with the sequence of the characters in the current string. The comparison returns results as positive, negative or 0 value. If there is a comparison between two strings and, if the first string is smaller than the second string, then it returns a negative result, if the first string is greater than the second string then, it results as a positive number and if both the strings are equal then, it returns zero.
Syntax
e.g
Output
concat()
The general meaning of concat is to add something. Same applies in Java too, concat() combines (concatenates) the string at the end of the specified string.
Syntax
e.g
Output
contains()
contains() searches for the specific characters in the string and if the sequence of characters are found, then it returns true, else it returns false.
Syntax
e.g
Output
endsWith()
endsWith() checks whether the string ends with the given suffix, if it ends with the suffix which is mentioned then it returns true, otherwise it returns false.
Syntax
e.g
Output
equals()
equals() compares the two given strings on the basis of the content of the string, if the two given strings are equal then it returns true, otherwise it returns false.
Syntax
e.g
Output
equalsIgnoreCase()
It works the same as equals(), but the only difference is equals() doesn’t allow lowercase or uppercase, but in case of equalsIgnoreCase() it compares the content of the two strings irrespective of lowercase or uppercase. Just like equals(), equalsIgnoreCase() returns true if the string matches with the other string irrespective of lowercase or uppercase.
Syntax
e.g
Output