Threads in Java
In the previous blog we learned about the Collection Class EnumMap and its methods. If you want to learn more about it, visit Threads in Java. In this, we will go through the basic concept of thread, multithreading, etc.
What is Thread
Earlier there was no way or approach wherein we could do multiple tasks at a time in parallel. As only one task was used to get executed, it was time-consuming and hard to manage. In order to overcome this drawback Thread was introduced. Thread is a light-weight process or the smallest part of the process that allows the program to perform more effectively by running numerous jobs concurrently. All the tasks are executed without affecting the main process. For example, if there is some interruption due to some operation in one process, then only that particular process will be impacted, the rest will work without any impact of that affected process. Each thread in a process is independent because all the threads in the process have their own separate path of execution.
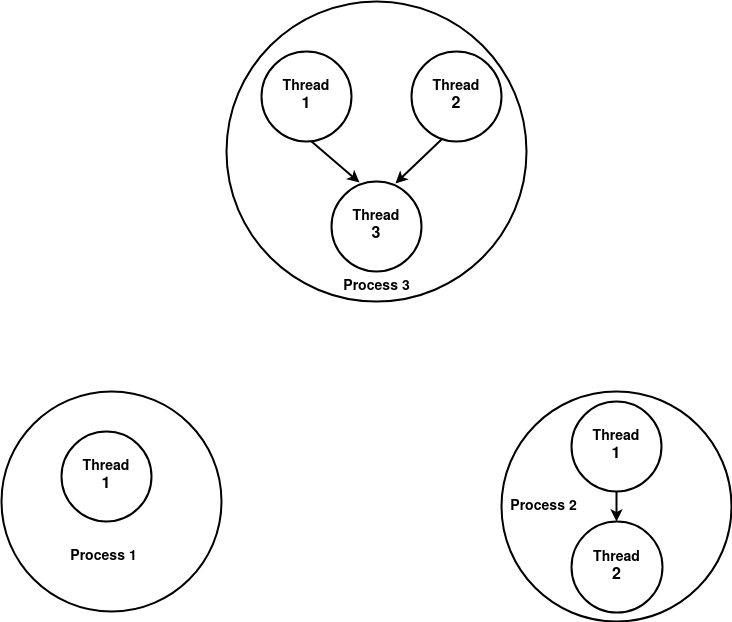
Life Cycle of Thread
The thread goes through various stages in the life cycle. The following are the stages:
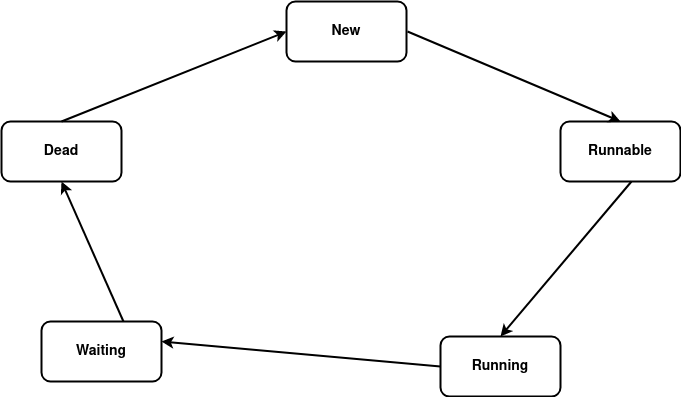
- New - In this stage, the thread is in a new state created by the Tread class. It will stay in this state till the program begins execution. It is also called a born thread.
- Runnable - In this stage, the thread is ready to execute the code. A new thread enters the runnable state when the start() method is called. In this stage, the thread is ready for executing the code and is waiting for the processor’s availability.
- Running - In this stage, the thread starts its execution. Meaning the CPU has allotted the thread a time slot for execution. The thread moves from a runnable state to a running state only when it is selected by the thread scheduler for execution.
- Waiting or Blocked State - When the thread is inactive for some time, then the thread enters a blocked or waiting state. For example, if there are two threads thread 1 and thread 2 where thread 2 wants to perform a printing operation and thread 1 is already performing a printing operation, then thread 2 has to wait until thread 1 completes its task. If we take another example with the same operation but now, in this case, both the threads have the same time slot allocated by the thread scheduler then, in that case, both the threads are in the blocked state.
- Terminated - The thread goes into the terminated state only for two reasons:
- When the thread finishes its execution of the task normally.
- Thread can terminate abnormally due to some unusual events like exceptions, faults, etc.
Creating a Thread
Thread can be created in two ways:
- By implementing a Runnable interface - In order to create a thread by implementing a Runnable interface, we need to first implement the run() method where we will put our business logic. Then create an object of the Thread class and call start()on that object.
- By extending the Thread class - In order to create a thread by extending a Thread class we need to first implement the run() method in which we will have our business logic. Then call the start() method.
Example of Thread by implementing Runnable Interface
Output
Example of Thread by extending Thread class
Output