TreeSet in Java
In the previous blog, we learned about HashSet in Java. If you want to know more about it, visit HashSet in Java. In this blog, we will learn about another Class in Collection Framework i.e. TreeSet. TreeSet is similar to HashSet, but the difference is, it is a sorted Collection. i.e. The elements inserted in random order will be presented in sorted order. For example, suppose we insert 4 elements in random order and we visit the elements. Then the values of those elements will be displayed in sorted order. Let's go ahead and take a brief look into it.
TreeSet
As the name suggests the sorting in the TreeSet is achieved by a tree data structure. Elements are stored in sorted order. TreeSet inherits AbstractSet class and implements the NavigableSet interface.
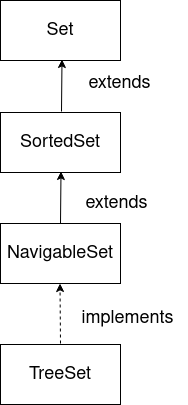
Syntax of Creating a TreeSet
Before creating a TreeSet, it is mandatory to import the package named java.util.TreeSet. If the package will not be imported then the compiler will give an error. So it is must to import the above package. Once the package is imported, we can create a TreeSet.
Syntax
Methods of TreeSet
- add()
- addAll()
- remove()
- removeAll()
- iterator()
- first()
- last()
- higher()
- lower()
- ceiling()
- floor()
- pollFirst()
- pollLast()
- headSet()
- tailSet()
add() method adds the specified element to the set if it is not present already.
e.g.
Output
addAll() method is used to all elements of the specified Collection to the set.
e.g.
Output
remove() method is to remove or delete the specified element from the set if it is present.
e.g.
Output
removeAll() method is used to remove or delete all the elements from the set.
e.g.
Output
iterator() method is used to access the elements of the TreeSet. But in order to use the iterator() we must import a package named java.util.Iterator.
e.g.
Output
first() method returns the first element of the set.
e.g.
Output
last() method returns the last element of the set.
e.g.
Output
higher() method returns the least element among those elements that are greater than the specified element.
e.g.
Output
lower() method returns the greatest element among those elements that are less than the specified element.
e.g.
Output
The ceiling() method returns the smallest element in this set that is bigger than or equal to the provided element, or null if no such element exists.
e.g.
Output
The floor() method returns the equal or nearest least element in the set to the provided element, or null if no such element exists.
e.g.
Output
pollFirst() method returns and removes the first element from the set.
e.g.
Output
pollLast() method returns and removes the last element from the set.
e.g.
Output
headSet() method returns all the elements of a TreeSet before the specified element. If the value true is passed to the method, then the method returns all the elements before the specified element including the specified element. The default value is false.
e.g.
Output
tailSet() method returns all the elements of a TreeSet after the specified element. If the value false is passed to the method, then the method returns all the elements after the specified element including the specified element. The default value is true.
e.g.
Output