Types of Exceptions in Java
In the previous blog, we learned about what is an exception handling. If you want to learn about it visit Exception Handling in Java . In this blog, we will go through the types of Exceptions.
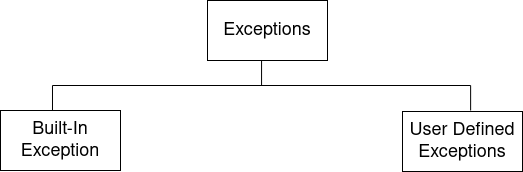
There are two types of Exceptions
- Built-in Exception
- User-defined Exception
- Built-in Exceptions
- ArrayIndexOutOfBoundsException
- ArithmeticException
- ClassNotFoundException
- FileNotFoundException
- IOException
- NoSuchMethodException
- NullPointerException
- NumberFormatException
- StringIndexOutOfBoundsException
- IllegalArgumentException
- IllegalStateException
- User-defined Exceptions
Built-in Exceptions are the exceptions that are already available in the Java libraries. Following are the built-in exceptions:
The exception ArrayIndexOutOfBoundsException is thrown when we attempt to access the array with an invalid index. For example, the size of an array is 4 and we are trying to access the 5th element which is not present in the array as the size is 4. In this case, the compiler throws an exception i.e.ArrayIndexOutOfBoundsException
e.g.
Output
ArithmeticException is an exception that is thrown when an exceptional arithmetic condition occurs. For example when we try to divide a number by 0 then in this case the compiler gives an exception.
e.g.
Output
ClassNotFoundException is the exception that is thrown when we try to access the class whose definition is not found. For example, we are trying to access the Class named Atrowel which is not present, so in this case, the compiler will throw an exception,i.e. ClassNotFoundException.
e.g.
Output
FileNotFoundException is an exception that is thrown when a try is not accessible or does not open. For example, we try to access a file named Example.txt and that file doesn’t exist then the compiler throws FileNotFoundException.
e.g.
Output
IOException is the exception that is thrown when there is a failure in input/output operation or if there is an interrupt due to some reason, then the compiler throws an exception called IOException. For example, if we have given a print command to a Printer and Printer has started printing pages and in between Printer goes out of Page, then the compiler throws an exception called IOException.
e.g.
Output
NoSuchMethodException is an exception that is thrown when we try to access the method which is not found. For example, suppose we try to access a method called displayResult and that method is not present in the class, then in this case the compiler will throw an exception i.e. NoSuchMethodException.
e.g.
Output
The NullPointerException exception is thrown when the program attempts to use an object reference that has a null value. For example, if we try to use the exObj reference which has the null value then the compiler throws an exception called NullPointerException.
e.g.
Output
NumberFormatException is an exception that is thrown when a program tries to convert a string with improper format into numeric values.
e.g.
Output
StringIndexOutOfBoundsException is thrown when the index of a string is either negative or greater than the length of the string. For example, suppose the length of an string is 20 and we are trying to access the 22th element then in this case the compiler will throw an exception i.e. StringIndexOutOfBoundsException.
e.g.
Output
IllegalArgumentException is an exception that is thrown when a method receives an argument that is improper or not accurate for the given condition, then the IllegalArgumentException is thrown. For example, if suppose there are two numbers and division needs to perform, and if one number from these two is zero then it will throw the exception i.e. IllegalArgumentException.
e.g.
Output
IllegalStateException is an exception that is thrown when a method is not accessible for the specific operation which we are performing.
e.g.
Output
In this example we are calling remove() before the next() method of iterable will throw an exception called IllegalStateException.
In Java we can define our own exceptions and throw them with the help of throw keyword. These exceptions are known as custom exceptions or user-defined exceptions. In order to create our own exception we should create an Exception class which will be the subclass for the Exception class. Note we should always extend the Exception class whenever we create our Exception class. For example:
e.g.
Once we have defined our exception, we can also create a default constructor which is as follows:
e.g.
We can even create a parameterized constructor by passing a string as parameters so that we can use this string parameter to store the details of exceptions. Once this is done we can call the superclass constructor and pass the string in that.
e.g.
In order to call this Exception we should create an object of it and throw it using the throw keyword.
e.g.
We will go through the program which illustrates the User-defined Exception
e.g.
Output