Global Object in JavaScript
In the null and undefined in Javascript blog, we have learned about the difference between null and undefined in JavaScript. Null signifies a deliberate absence of value, commonly used in programming. On the other hand, undefined implies a deeper absence, often linked to uninitialized variables or non-existent properties. Now, let's explore the Global Object in JavaScript.
What is the Global Object in JavaSript?
The Global Object provides variables and functions accessible from anywhere in our program. Properties of the global object can be used directly without the need for importing. For instance, we can usealert or console.log directly. We can even add our own properties. Consider the example:
We can define our own properties, as shown in the above example:
In the above example, many will think it will display the message“Welcome” in the alert box. On the contrary, it will display the message “Welcome” in the console, as the alert property is overridden by the console log. Take a look at the screenshot below
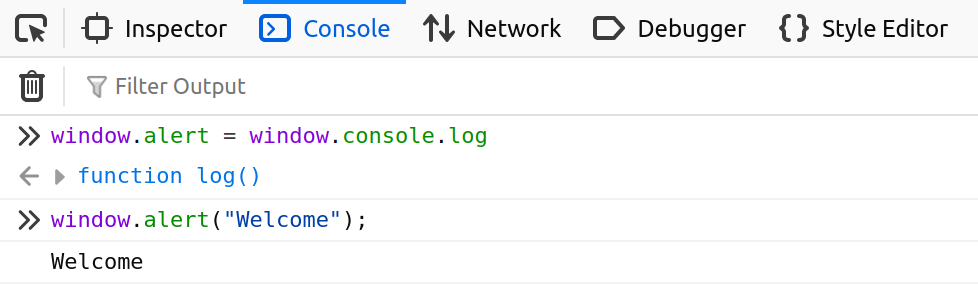
Global Objects in different Platforms
In browsers, the window serves as the global object, while Node.jsuses global. Other environments may have different names. Here, we will be using a window object as we are using a browser. In order to display the window object, we can display it using a number of properties. For example, we can use window, this, self, globalThis, etc. Let's see it with the help of an example:
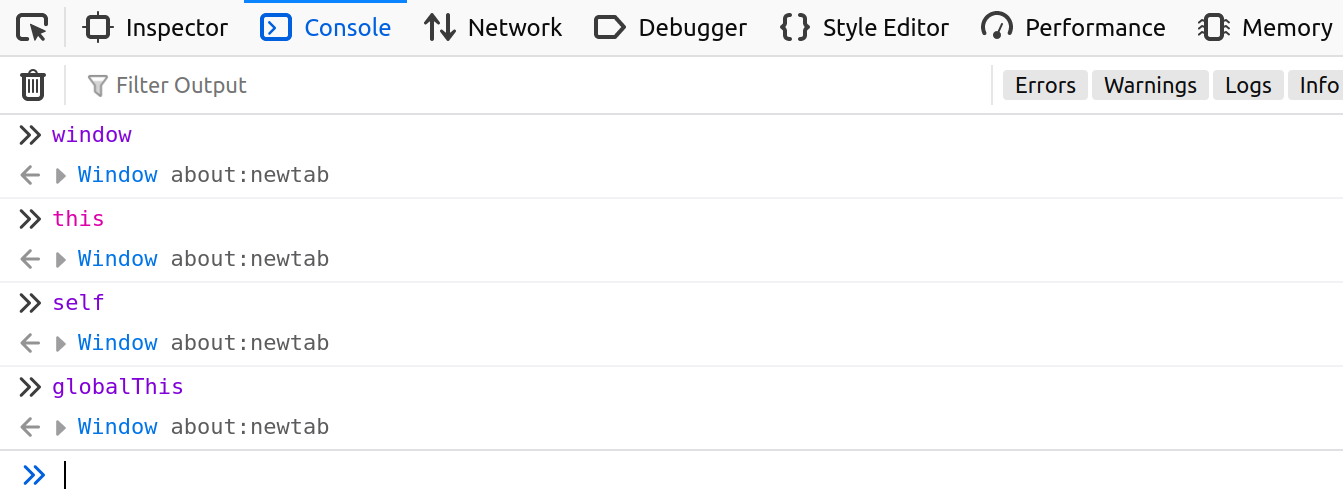
In the above screenshot, we have used different properties to display the window object. But there may be cases where some of the properties mentioned above may not be supported by the other browser, like for example, self, which may not be supported in safari, etc. So in order to avoid this situation, globalThis is added to the language, which is supported in almost all the browsers.
Optimizing Global Property
In order to make use of global property, let’s take an example. Now let’s say I want to display some text globally, so this is how it can be done.
In the above example, we have a displayMsg variable that displays the message. With the help of the var keyword, we can access the variables and functions from anywhere. As var is considered a global variable, whatever we define with var can be accessed globally. For example, let's see the output of the above statement.
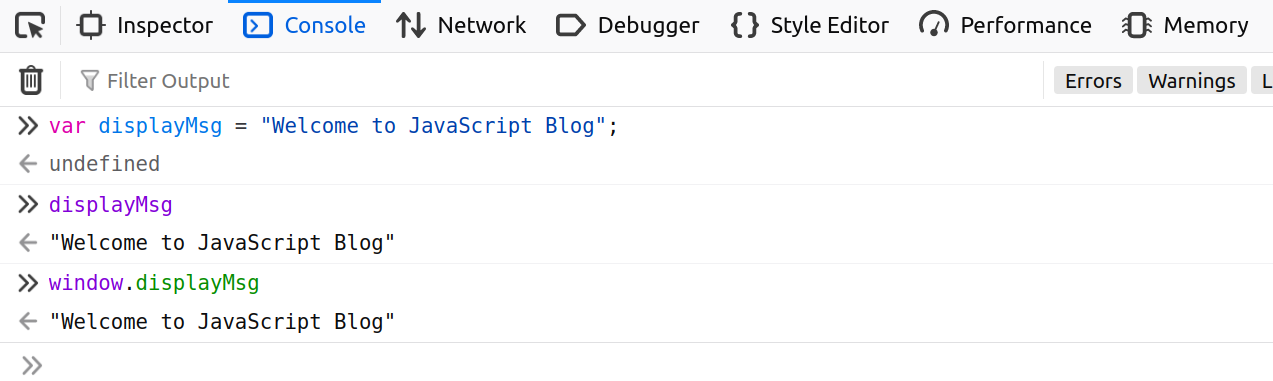
In the above screenshot, we can see that the message can be displayed in two ways. First by simply using the variable name, and second by using a window object. This is because the var's scope is global; we will see the variable present in the window object as well. We can even get the custom function that we have defined. Let see with the help of example
In the above function, we have used alert to display the message. So now let's take a look at its output.
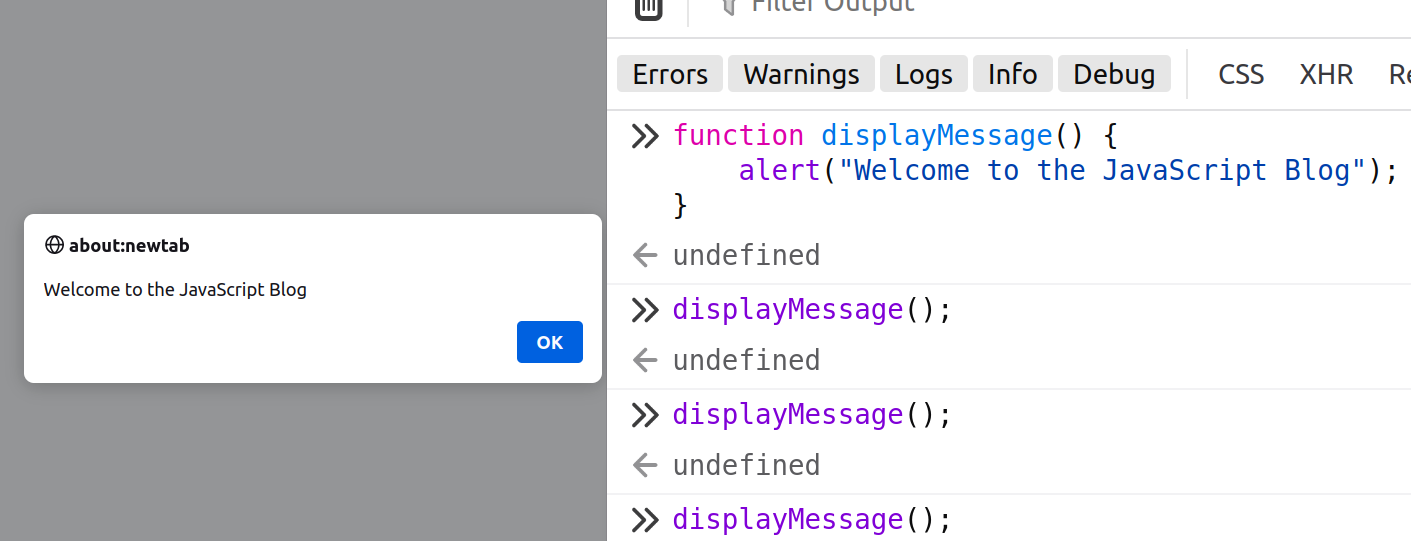
In the above screenshot, we can see that we have just called the function in the console, and we have the alert box containing the message. Now the same function can be accessible using window objects as well. Let take a look
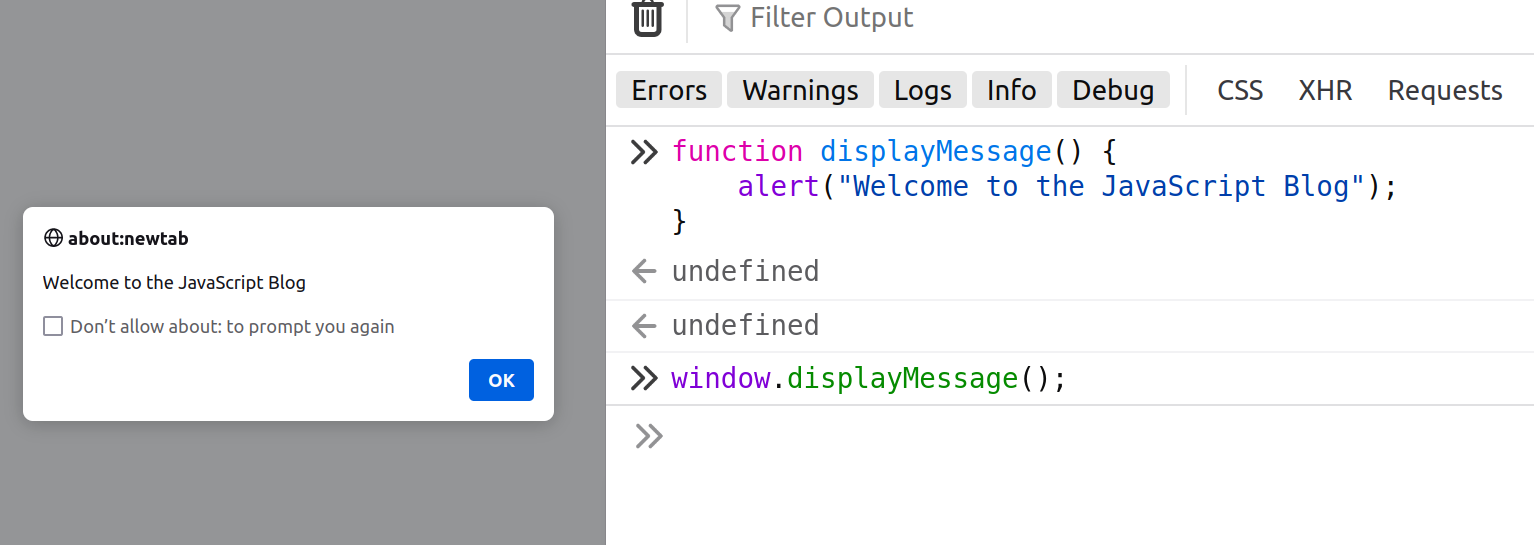
Now the question may arise that if we define a variable with varinside the function, then can this variable be accessible globally?. So the answer for this is no because we can access only those variables that we have defined before the function; we cannot access the variables that are defined inside the function as that variable’s scope will be only inside its function.
The Global Object in JavaScript acts as a hub for essential variables and functions accessible throughout our code. Understanding and leveraging this powerful tool enhances the efficiency of our programming.