Reverse a String in JavaScript
In Strings in JavaScript blog, we have learned about Strings, its methods, etc. In JavaScript, strings are sequences of characters. String methods like toUpperCase() and indexOf() help manipulate and search within strings. Understanding and using these methods enhances string handling in our code, making it more efficient and versatile. In this blog, we will learn about how we can reverse a string in JavaScript.
Reverse a String
In JavaScript, reversing a string is possible in various ways, which we will be learning one by one. Before taking a look into it, let's first understand how the reverse string looks like with an example
String1: Welcome to JavaScript Blog
Reversed String: golB tpircSavaJ ot emocleW
String2: Reverse a String in JavaScript
Reversed String: tpircSavaJ ni gnirtS a esreveR
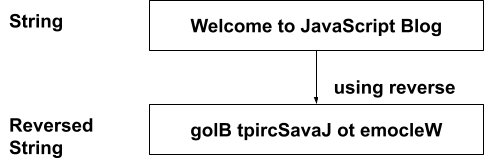
Approach 1: Using for loop
In this approach, a for loop is used to iterate through the characters of the string in reverse order. It begins from the last character, i.e., str.length - 1, and the loop decrements i until it reaches 0. In each step, the current character, i.e., str[i] is added to the reversed string. Let’s take a look at the example
Output
Approach2: Using split(), reverse() and join() Methods
In this approach, we use three steps to reverse a string. First, thesplit() method breaks the string into an array of characters. Then, the reverse() method flips the order of the array. Finally, thejoin() method merges the reversed characters back into a string, essentially reversing the original one. Let’s understand it graphically
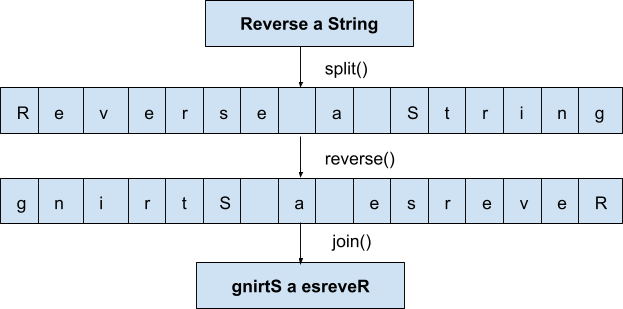
Let’s take a look at the example
Output
Approach3: Using Spread Operation
In this approach, the spread operator ([...str1) is used to convert the string into an array of characters. Then, the reverse() method is applied to reverse the order of the characters, and join('')combines them back into a string. The result is a reversed version of the original string. Let’s understand it graphically
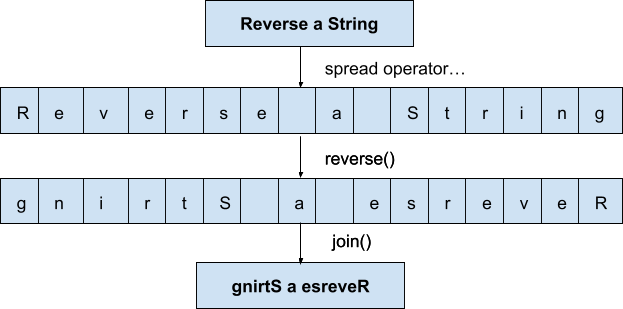
Let’s take a look at the example
Output
Approach4: Using for..of loop
In this approach, the for...of loop iterates through each character in the input string, and during each iteration, the character is added to the beginning of the reversed string. This process effectively reverses the original string. Let’s understand it graphically
Original String: JavaScript
Reversed String: “”
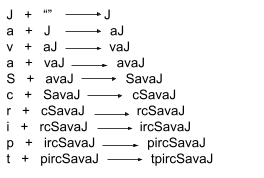
Original String: JavaScript
Reversed String: tpircSavaJ
Let’s take a look at the example
Output
Approach5: Using substring() and decrementing index
In this approach, we use the substring() method to pick each character at index i and add it to the reversed string. After that, we decrease the index i. Let’s take a look at the example
Output
Approach6: Using recursion
In this approach, the recursive approach keeps calling itself. It takes the substring starting from the second character and adds it to the first character. This process continues until it reaches the base case, effectively reversing the string. Let’s take a look at the example
Output
In JavaScript, reversing a string can be achieved through various methods, such as using loops or recursion. Employing a for loop or the spread operator provides straightforward solutions. Additionally, understanding the subtleties of string manipulation methods like substring() contributes to an efficient reversal process. Exploring these techniques enhances your coding capabilities, offering flexibility in handling strings for diverse applications.